Add lesser-known lodash gems to your toolbox - Objects
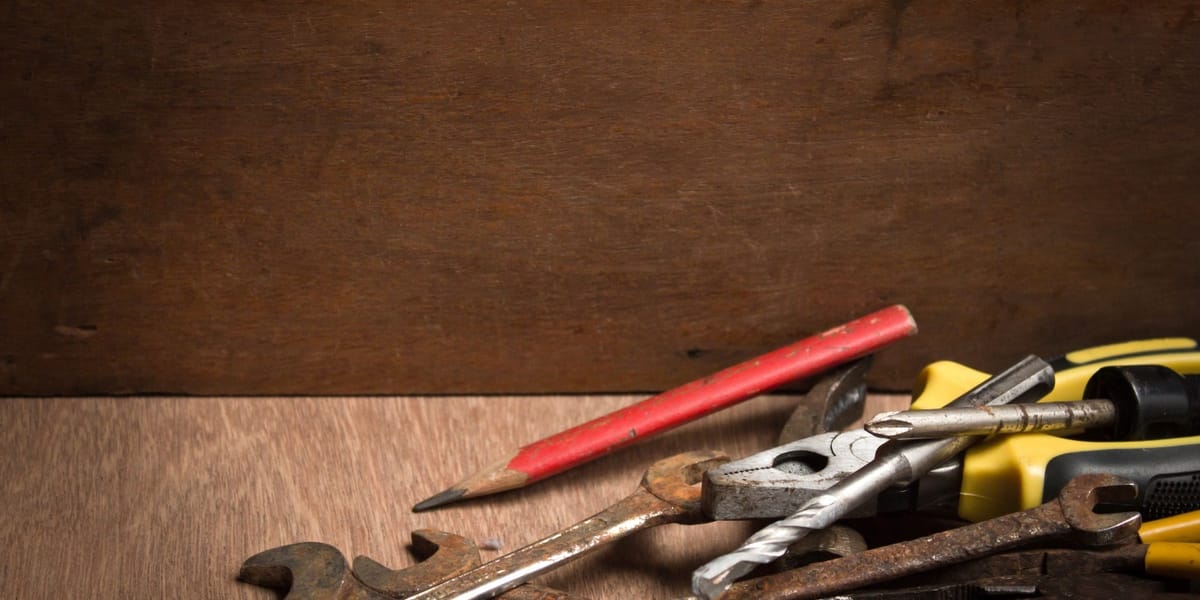
This is a part two of lodash series.
- Strings
- Objects
Removing falsey values from object
I asked from the community to share some lodash/underscore tips and this one got puzzled.
@_Tx3 Return a copy of an object with falsey values removed. _.pick(obj, _.identity);— Jezen Thomas (@jezenthomas) June 5, 2015
Embedded JavaScript
This means that if I'm using object literal like a hashmap:
techCompanies = {
nokia: {
www: 'nokia.com'
},
apple: undefined,
motorola: {
www: 'apple.com'
}
}
Then I can remove all falsey values from the object like this:
_.pick(techCompanies, _.identity);
> Object {nokia: Object, motorola: Object}
How does it work?
_.pick
takes an object as first argument and string/string[]/function as a second argument. Simplest use case could be using _.pick with string argument _.pick(techCompanies, 'apple')
and it would return apple object.
In the example we're using _.identity
as a second argument and it's a bit mind-confusing little function.
This method returns the first argument provided to it.
The sentence gets me thinking: where's the catch.
But it really is that simple. If null is passed it will return null, if number 2 is passed it will return number 2 and so on.
If _.pick
second argument is a function then it will call that function with each property value. It will pick all properties that had function returning true
!
Defaults using .assign
This is probably one the most common object related function in lodash and Underscore. With _.assign you can easily copy object properties.
You can have multiple source objects and the rightmost argument will override. Therefore the default value object is normally first source object.
/**
* Create widget to a dashboard.
* @param {Object} widget - The widget that displays beautiful data
* @param {string} widget.name - The name of the widget
*/
Dashboard.prototype.create(widget) {
var defaults = {
name: 'Default name'
};
widget = _.assign({}, defaults , widget);
}
Now when we call .create
without name we get default name.
Note: The first argument is destination, but I like to make the variable assign more explicit by using the return value
There is also a function called _.defaults that does this same thing, but with little bit different way of thinking.
_.defaults(widget, defaults);
Assigns own enumerable properties of source object(s) to the destination object for all destination properties that resolve to undefined. Once a property is set, additional values of the same property are ignored.
One thing to note is that widget
is mutated.
For some reason I prefer the first method, it might be just that I'm more used to it or I prefer immutability.
Don't miss commonly used functions such as: _.keys and _.values
What are your favourite object related use of lodash/underscore functions? I would be very happy if you'd share your wisdom to others.