Debugging Jest Tests in Visual Studio Code When Using WSL
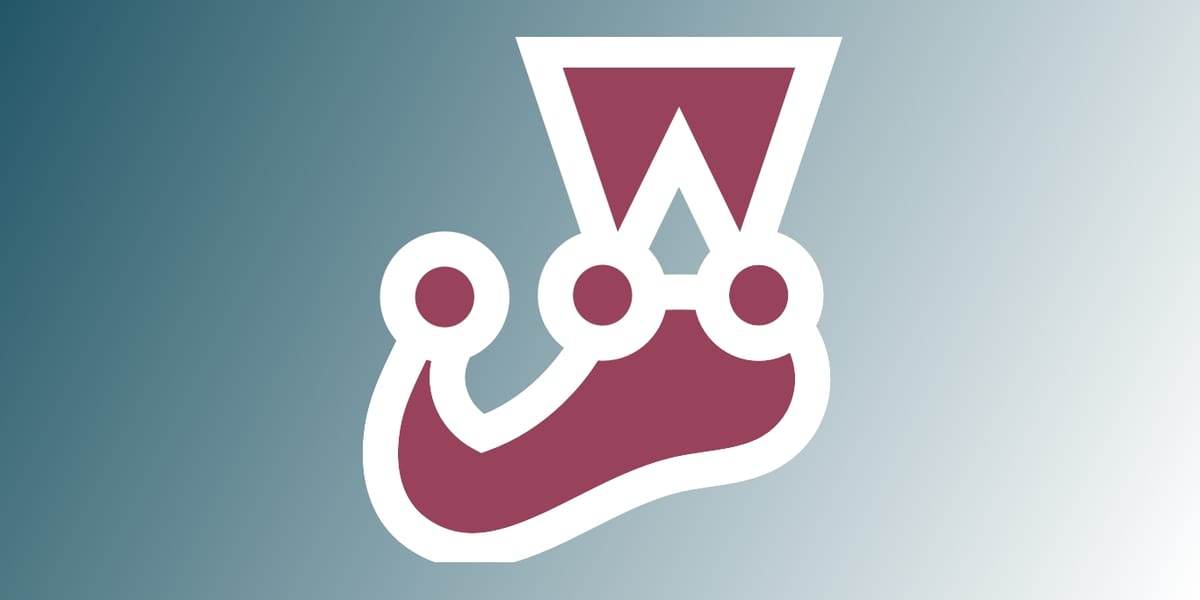
In the ideal world, investigating why a test fails should be a trivial task, but sometimes the code that is under testing is not easy to follow. Or, even the test can be hard to understand. To get understanding what is going on you can use console.log
in the tests, but it does become cumbersome if you don't know which variable value you want to investigate. In this blog post, I'll show how to configure Visual Studio Code as a debugging environment for Jest tests. As an extra, the text will cover the WSL (Windows Subsystem for Linux) configuration.
Finding the launch configuration
Visual Studio Code has a Debug section where all the breakpoints, available variables, statement watches are listed.
You can start debugging by hitting F5 or play button. In the screenshot, there is a debug configuration active for launching a Chrome instance.
To add a new configuration for Jest, hit the cog on the right side of the Launch Chrome selection. It will open a new tab with file launch.json.
Or, you can open command palette and search for "Debug: Open launch.json".
The configuration file (launch.json)
Most likely the launch.json is not empty, and you might find something like this in the file:
{
"version": "0.2.0",
"configurations": [
{
"type": "chrome",
"request": "launch",
"name": "Launch Chrome",
"url": "http://localhost:3000",
"webRoot": "${workspaceFolder}",
"smartStep": true
}
]
}
To add new launch configuration for Jest, add a new block:
{
"type": "node",
"request": "launch",
"name": "Jest All",
"program": "${workspaceFolder}/node_modules/jest/bin/jest",
"args": ["--runInBand"],
"useWSL": true
}
This configuration works on a Windows machine that has the application installed in WSL, for example, in the Ubuntu shell the command npm install
has been executed.
Let's go through all the config parameters.
type
, is node as we're not running in the browser (see the other launch config with the Chrome debug).
request
, as I am writing this there are two options: launch and attach. We're launching a new debugging session, so we're using the launch option.
program
, points to the executable. This parameter can vary depending on which platform you're running. See Debugging in VS Code section of Jest documentation.
The explanation for the runInBand argument:
Note: the --runInBand cli option makes sure Jest runs test in the same process rather than spawning processes for individual tests. Normally Jest parallelizes test runs across processes but it is hard to debug many processes at the same time. Tests are failing and you don't know why - Jest Documentation
useWSL
enables Windows Subsystem for Linux support.
Debugging the tests
Adding a breakpoint is easy, click next to the line number to add one. You might have customized the view, so the line number could be hidden or on the right side of the text, but adding a breakpoint should be still possible by clicking to the side of the text editor.
The added breakpoint can also be seen seen on the Debug panel.
To start debugging, open debug panel, choose the right configuration and press the green play symbol.
After a while, you should see the line being active where you added the breakpoint.
Debug Console view, which can found using Ctrl + Shift + Y, contains information on how the debugger attached to the testing process. That view is shown in the right side of the screenshot above.
Once you have a debugging session active you can check the value of the variable by moving the mouse cursor on top of the variable, see available variables in various scopes (Variables section in the Debug panel.) etc.
I hope this will help you get started with unit test debugging!